Using JavaScript to embed a YouTube video
This example uses JavaScript to create an iframe and play the YouTube video on click of the anchor.
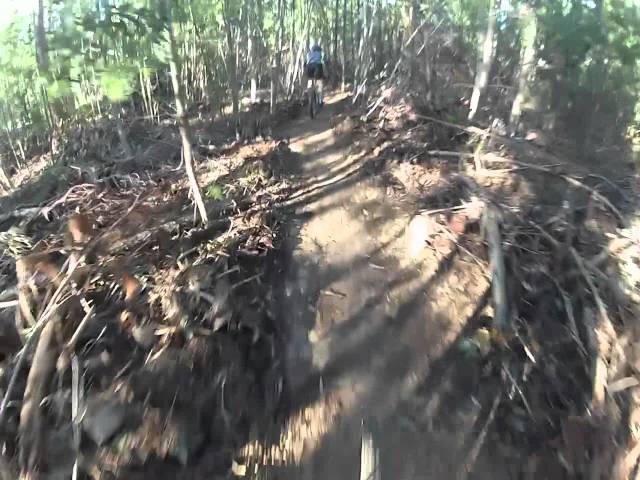
HTML
<figure class="figure">
<a class="js-embed-iframe" href="https://www.youtube.com/embed/yPowHRjSFCA?autoplay=1&rel=0" target="_blank">
<img src="http://img.youtube.com/vi/yPowHRjSFCA/sddefault.jpg" width="640" height="480" alt="Cover image of video (standard definition)">
</a>
<figcaption>Die Burger 42km MTB Challenge 2012 [Part 9 of 11] [GoPro HD Hero2]</figcaption>
</figure>
JavaScript
<script>
// Get all iframes to be embedded
var embeds = document.querySelectorAll('.js-embed-iframe');
embeds.forEach(function (embed) {
// Get the href from the anchor for each iframe to be embedded
var url = embed.getAttribute('href');
// Check that there is a URL
if (typeof url !== 'undefined' && url !== '') {
// Add an on click event listener to the anchor
embed.addEventListener('click', function (e) {
var anchor = this;
// Prevent clicking through to the href
e.preventDefault();
// Create the iframe and set the source attribute to the value of the anchor’s href
var iframe = document.createElement('iframe');
iframe.setAttribute('src', url);
// Get the parent of the anchor and replace the anchor with the iframe + remove the figure’s caption
var parent = anchor.parentNode;
parent.replaceChild(iframe, anchor);
parent.removeChild(document.querySelector('figcaption'));
});
}
});
</script>
Read about the default method for embedding a YouTube video.
Or go back to the performance comparision of these two methods.